Selenium WebDriver: Dynamic Element Handling
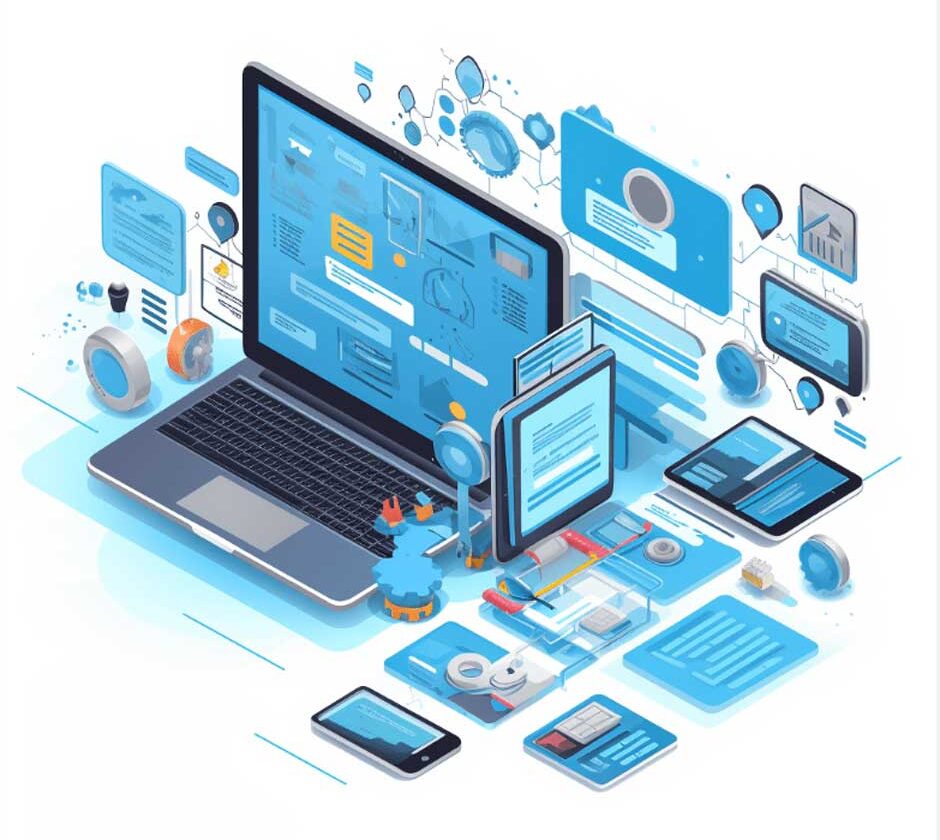
In modern web applications, elements are sometimes dynamic. Elements may load dynamically based on user actions, like clicking a button, or may appear after a delay, making them challenging to handle in automated tests. Using Selenium WebDriver, you can tackle these dynamic elements by waiting for them to appear, disappear, or meet specific conditions before interacting with them. This approach makes your tests more reliable and helps reduce test failures due to timing issues.
In this blog, we’ll explore strategies to handle dynamic elements effectively using Selenium WebDriver, with clear examples to help you make your tests robust and adaptive.
Read to know more about what is Selenium WebDriver?
What is Selenium WebDriver?
Selenium WebDriver is a powerful tool for automating web browser interactions, making it a favorite among testers and developers for creating reliable, repeatable test scripts.
Unlike traditional record-and-playback tools, WebDriver directly communicates with the browser, simulating user actions like clicking buttons, filling out forms, and navigating between pages. This direct interaction means tests can run across multiple browsers and platforms with ease, providing a flexible approach to web testing.
Designed with modern web applications in mind, Selenium WebDriver supports dynamic content, making it ideal for testing today’s interactive and responsive websites. It’s an essential tool for anyone looking to automate repetitive tasks, improve test accuracy, and streamline the quality assurance process.
What are Dynamic Elements in Selenium?
Dynamic elements in Selenium are elements on a webpage with attributes that change during runtime. These elements might have different IDs, classes, names, or other properties that vary when the page loads or updates. This variability can create challenges for automation testing, as standard methods of identifying elements may not be reliable.
To ensure accurate interaction and validation during test execution, test scripts must be designed to effectively manage these changing elements. You can handle dynamic elements in Selenium more accurately using techniques like explicit waits and unique identifiers.
How to Handle Dynamic Web Elements?
There are many ways to handle dynamic elements, so let’s discuss them with code examples.
1) Explicit Waits
Explicit waits are among the most effective strategies for handling elements that load with delays in Selenium. By using explicit waits, you can direct Selenium to pause test execution until specified conditions are met, thereby improving test stability and minimizing timing issues. The ExpectedConditions class in Selenium provides a variety of conditions to wait for, such as an element becoming clickable, visible, or present in the DOM. This approach gives you precise control over your interactions, allowing you to specify the exact state an element must reach before the test proceeds.
Explicit waits are particularly valuable when testing dynamic web pages where elements may load or change based on user interactions, ensuring that your test advances only when the required element is fully ready.
Example:
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement dynamicElement = wait.until(ExpectedConditions.elementToBeClickable(By.id(“dynamicElementId”)));
2) Fluent Waits
Fluent waits offer enhanced flexibility in Selenium by allowing you to set custom polling intervals and specify which exceptions to ignore while waiting for an element. This approach is particularly useful for handling elements that may take unpredictable amounts of time to load or appear due to dynamic content or network conditions.
With fluent waits, you can define the maximum wait time, set regular polling intervals to check for the element, and specify certain exceptions, such as `NoSuchElementException,` to be ignored during the wait period. This fine-tuned control helps ensure your tests adapt to varying load times, making them more robust and reliable, especially in environments where response times fluctuate.
Example:
Wait wait = new FluentWait(driver)
.withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofSeconds(2))
.ignoring(NoSuchElementException.class);
WebElement dynamicElement = (WebElement) wait.until(driver -> driver.findElement(By.id(“dynamicElementId”)));
3) CSS Selectors and XPath
When working with dynamic web elements whose attributes change frequently, you can use CSS selectors or XPath to locate these elements based on stable attributes. This approach allows you to target elements more reliably. For instance, instead of depending on an element’s ID (which may vary with each load), consider locating it by its class name or data attributes, which are often consistent and provide better stability.
WebElement dynamicElement = driver.findElement(By.cssSelector(“.dynamic-class”));
4) Handling Elements Inside Frames
When handling elements located within iframes, you need to switch to the iframe’s context before interacting with any elements inside it. Switching context ensures that Selenium can effectively locate and interact with these elements. Without switching to the iframe, any actions targeting elements within it will fail, as Selenium remains in the main document context by default.
Example:
driver.switchTo().frame(“iframeName”);
WebElement dynamicElement = driver.findElement(By.id(“elementInFrame”));
5) Using Relative Element Positions
In some cases, you can anticipate the position of a dynamic element in relation to a nearby stable element. By leveraging XPath, you can locate the dynamic element based on its proximity to this stable reference. This technique can be particularly effective for elements that consistently appear near a more predictable element, providing a dependable anchor point for your locator strategy.
6) Multiple Attributes
If a single attribute isn’t enough to uniquely identify a dynamic element, you can combine multiple attributes to create a more reliable locator. Using logical operators like and or or in your XPath or CSS selectors lets you target elements based on several attribute values.
For example, you might use an XPath to locate a button with an ID that starts with a specific string and also has a certain class. This multi-attribute approach makes your locator more precise, ensuring the dynamic element is consistently and uniquely identified, even if its attributes change.
7) Refresh the Page
In some cases, refreshing the page may be a quick workaround for dealing with dynamic elements, especially if they appear after a page reload.
driver.navigate().refresh();
8) Retrying Mechanism
Consider implementing a retry mechanism where Selenium repeatedly attempts to locate and interact with the element until a specified timeout is reached. This approach is especially useful when you expect delays in the element’s appearance, allowing Selenium to keep trying until the element becomes available. By combining retries with an appropriate timeout, you can enhance the reliability of interactions with dynamic elements that may take time to load.
Example:
boolean isDynamicElementPresent = false;
int attempts = 0;
WebDriverWait wait = new WebDriverWait(driver, 10); // Adjust the timeout as needed
while (!isDynamicElementPresent && attempts < 3) {
try {
dynamicElement = wait.until(ExpectedConditions.presenceOfElementLocated(By.id(“dynamicElementId”)));
isDynamicElementPresent = true;
} catch (NoSuchElementException e) {
attempts++;
}
}
LambdaTest is an AI-powered testing platform and is at the forefront of the Selenium testing revolution, combining the power of artificial intelligence with cutting-edge technology to deliver unparalleled testing capabilities.
Key Features of LambdaTest for AI testing:
- Flaky Test Detection: LambdaTest uses AI to identify flaky tests that produce inconsistent results, helping teams focus on resolving root causes efficiently.
- Smart Test Execution: The platform optimizes test execution by identifying and prioritizing the most critical test cases using machine learning, reducing testing time and resource consumption.
- AI-Driven Debugging: Leverage AI-assisted insights to pinpoint and resolve bugs faster with advanced analytics and visual logs.
- Cross-Browser and Cross-Device Testing: Automate tests across thousands of browser and device combinations while benefiting from AI insights to improve test coverage.
- Performance and Load Testing: AI identifies patterns and bottlenecks, offering actionable recommendations to enhance application performance.
- Seamless CI/CD Integration: Accelerate your DevOps pipeline with intelligent test orchestration and real-time feedback on test performance.
End Note
Selenium dynamic elements refer to web page elements with attributes or properties that can change at runtime. You can use strategies like XPath and CSS selectors with dynamic classes or IDs to locate dynamic aspects.
You can also explicitly use relative element positions, waits, and patterns for dynamic element identification. The absolute path method uses the full XPath, starting at the root. However, it is not recommended because the website structure may change.
Relatives that begin or contain text allow for the location of elements based on attribute-value patterns. This flexibility is useful for handling dynamic aspects. When multiple elements share the same locator, it is helpful to identify the element by index. The index can then find the desired element.
Combining different attribute values in locators will enhance specificity. To handle dynamic elements, you must analyze their behavior and identify patterns. Then, adapt the locator strategy accordingly.
Using the right techniques will ensure that dynamic elements are reliably identified and interacted with during Selenium automation tests.